JavaScript has become an integral part of modern web development, enabling dynamic and interactive features on websites. However, its increasing popularity has also made it a target for malicious actors seeking to exploit vulnerabilities in web applications. In this article, we will explore the security considerations surrounding JavaScript in web browsers and discuss best practices for writing secure code.
​
The Security Risks
While JavaScript itself is not inherently insecure, its execution within the web browser environment introduces certain risks that developers must be aware of. Here are some common security concerns associated with JavaScript:
​
1. Cross-Site Scripting (XSS)
XSS attacks occur when an attacker injects malicious code into a website, which is then executed by unsuspecting users. JavaScript is often used as the payload in XSS attacks, as it can manipulate the DOM and interact with sensitive user information. To mitigate this risk, developers should implement input validation and output encoding to prevent the execution of untrusted code.
​
2. Cross-Site Request Forgery (CSRF)
CSRF attacks exploit the trust that web browsers have in authenticated sessions. By tricking a user into performing unintended actions on a website, an attacker can manipulate their session and perform malicious actions on their behalf. To prevent CSRF attacks, developers should implement measures such as using CSRF tokens and validating the origin of incoming requests.
​
3. Insecure Direct Object References (IDOR)
JavaScript can expose sensitive data if developers do not properly secure access to resources. Insecure Direct Object References occur when an attacker can directly access internal object references, such as database IDs, and manipulate them to gain unauthorized access. Developers should implement proper authorization checks and avoid exposing internal references in client-side code.
​
4. Clickjacking
Clickjacking is a technique used to deceive users into clicking on hidden or disguised elements on a webpage. JavaScript can be used to manipulate the visibility and positioning of elements, making it easier for attackers to trick users into performing unintended actions. To prevent clickjacking, developers should implement frame-busting techniques and ensure that user interactions are explicitly authorized.
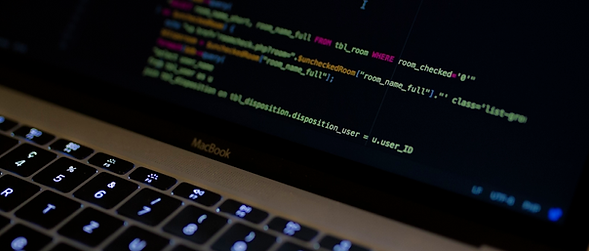
Best Practices for Writing Secure JavaScript Code
Now that we understand the potential security risks associated with JavaScript, let's explore some best practices for writing secure code:
​
1. Input Validation and Output Encoding
One of the most effective ways to prevent XSS attacks is to implement proper input validation and output encoding. Developers should validate and sanitize all user input to ensure that it does not contain malicious code. Additionally, any data that is output to the browser should be properly encoded to prevent unintended execution of JavaScript.
​
2. Use Strict Mode
Enabling strict mode in JavaScript helps catch common programming mistakes and enforces stricter rules for variable declaration and usage. This can help prevent accidental global variable leaks and improve code quality. To enable strict mode, add the following line at the beginning of your JavaScript file or script tag:
'use strict';
​
3. Avoid Inline Scripts
Inline scripts, where JavaScript code is directly embedded within HTML tags, should be avoided whenever possible. Instead, externalize your JavaScript code into separate files and include them using the <script> tag. This separation of concerns improves code maintainability and makes it easier to implement security measures.
​
4. Implement Content Security Policy (CSP)
Content Security Policy is a security mechanism that helps prevent the execution of malicious scripts by defining the sources from which the browser can load resources. By implementing a strong CSP, developers can reduce the impact of XSS attacks and enforce stricter security policies. It is recommended to use a whitelist approach, specifying only trusted sources for scripts, stylesheets, and other resources.
​
5. Regularly Update Dependencies
JavaScript libraries and frameworks often release security patches and updates to address known vulnerabilities. It is crucial to regularly update your dependencies to ensure that you are using the latest, most secure versions. Additionally, consider using tools like Snyk or npm audit to scan your codebase for known vulnerabilities.
​
6. Implement Access Controls
To prevent Insecure Direct Object References (IDOR) and other authorization-related vulnerabilities, it is important to implement proper access controls. Ensure that sensitive resources are protected by authentication and authorization mechanisms, and avoid exposing internal references or sensitive information in client-side code.
​
7. Use HTTPS
Securing the communication between the web browser and the server is essential for protecting user data and preventing man-in-the-middle attacks. Always use HTTPS to encrypt the data transmitted between the client and the server, ensuring the confidentiality and integrity of sensitive information.
​
8. Regularly Test and Audit Your Code
Performing regular security testing and code audits is crucial for identifying and addressing potential vulnerabilities in your JavaScript code. Use tools like static code analyzers, security scanners, and penetration testing to identify any weaknesses and ensure that your code adheres to best practices.
​
Conclusion
JavaScript is a powerful tool for creating dynamic and interactive web experiences, but its execution within web browsers introduces security risks that developers must address. By understanding the potential vulnerabilities and following best practices for writing secure code, developers can minimize the risk of JavaScript-related security issues and protect their users' data and privacy. Learn more about security on secureprivateinformation.com